OAuth 2.0 with Gmail over IMAP for web applications (DotNetOpenAuth)
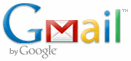
OAuth is an open protocol to allow secure API authorization in a simple and standard method from desktop and web applications.
This article describes using OAuth 2.0 to access Gmail IMAP and SMTP servers using .NET IMAP component in web application scenario (ASP.NET/ASP.NET MVC). You can also use OAuth 2.0 for installed applications.
DotNetOpenAuth
First download the latest version of DotNetOpenAuth – it’s free, open source library that implements OAuth 2.0: http://www.dotnetopenauth.net
Add it as a reference and import namespaces:
// c#
using DotNetOpenAuth.OAuth2;
using DotNetOpenAuth.OAuth2.Messages;
using DotNetOpenAuth.Messaging;
' VB.NET
Imports DotNetOpenAuth.OAuth2
Imports DotNetOpenAuth.OAuth2.Messages
Imports DotNetOpenAuth.Messaging
Register Application
Before you can use OAuth 2.0, you must register your application using the Google Developers Console. After you’ve registered, go to the API Access tab and copy the “Client ID” and “Client secret” values and specify “Redirect URI“, which you’ll need later.
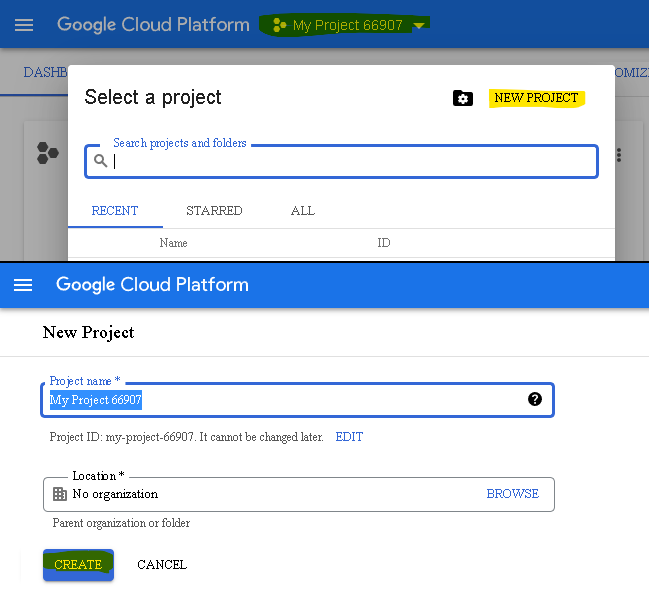
At least product name must be specified:
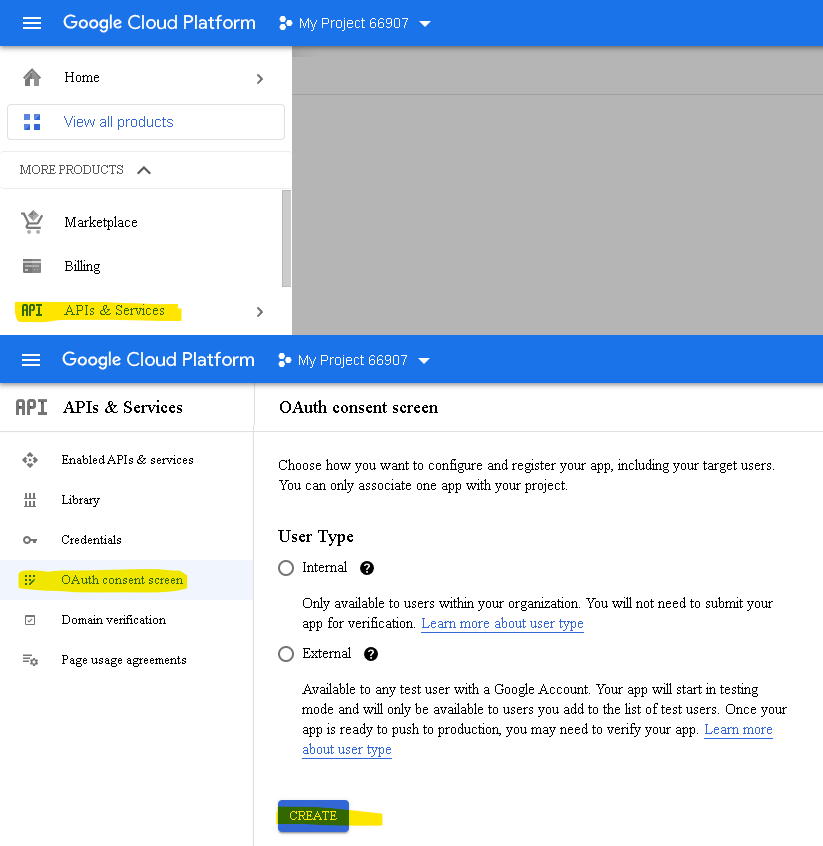
Now create credentials:
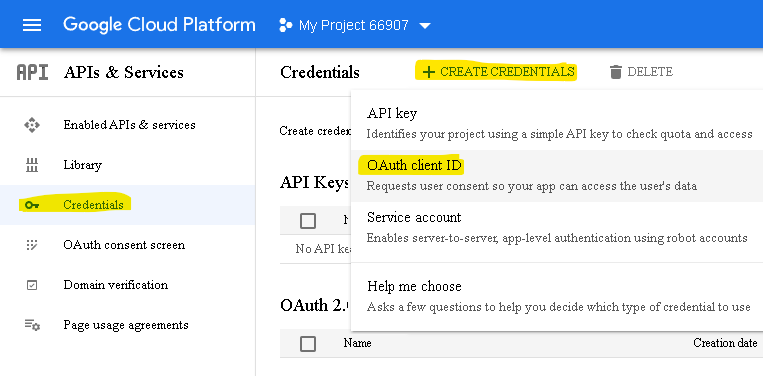
Specify redirect URI:
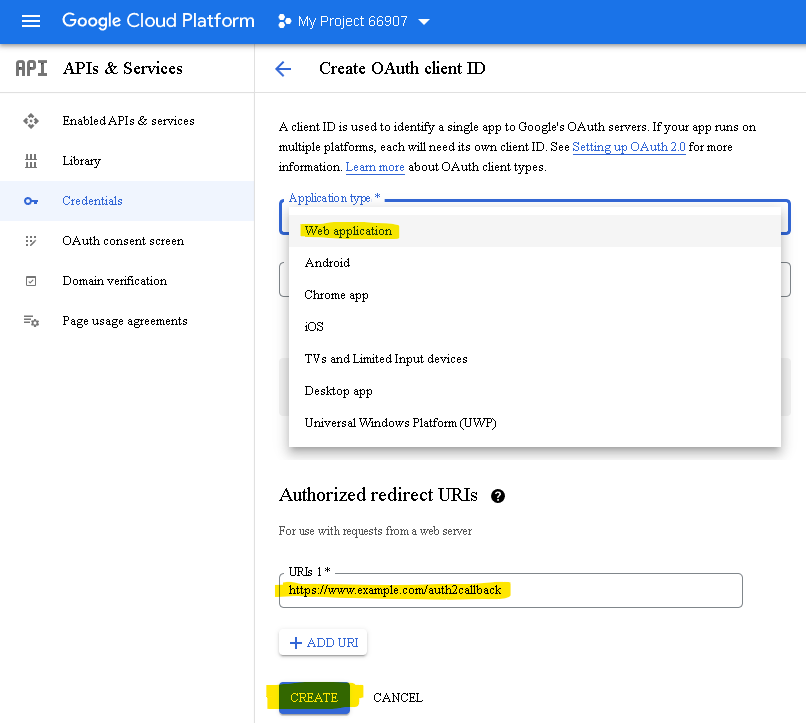
After you’ve registered, copy the “Client ID” and “Client secret” values, which you’ll need later:
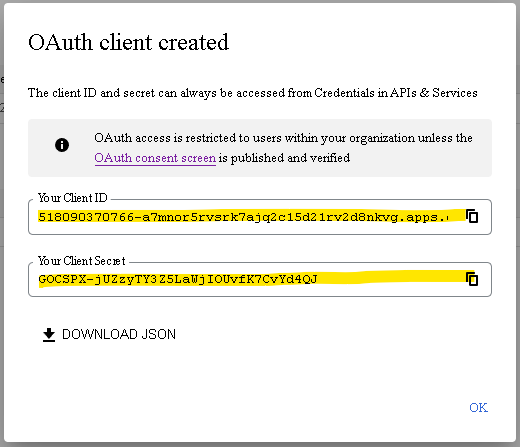
Now we can define clientID, clientSecret, redirect url and scope variables, as well as Google OAuth 2.0 server addresses. Scope basically specifies what services we want to have access to. In our case it is user’s email address and IMAP/SMTP access:
const string clientID = "12345.apps.googleusercontent.com";
const string clientSecret = "XXXYYY111";
const string redirectUri = "http://www.yourdomain.com/oauth2callback";
AuthorizationServerDescription server = new AuthorizationServerDescription
{
AuthorizationEndpoint = new Uri("https://accounts.google.com/o/oauth2/auth"),
TokenEndpoint = new Uri("https://oauth2.googleapis.com/token"),
ProtocolVersion = ProtocolVersion.V20,
};
List<string> scope = new List<string>
{
GoogleScope.ImapAndSmtp.Name,
GoogleScope.UserInfoEmailScope.Name
};
Obtain an OAuth 2.0 access token
As we are using ASP.NET we’ll use WebServerClient class:
WebServerClient consumer = new WebServerClient(server, clientID, clientSecret);
// Here redirect to authorization site occurs
consumer.RequestUserAuthorization(scope, new Uri(redirectUri));
If you use ASP.NET MVC the last line is different:
// Here redirect to authorization site occurs
OutgoingWebResponse response = consumer.PrepareRequestUserAuthorization(
scope, new Uri(redirectUri));
return response.AsActionResult();
At this point user is redirected to Google to authorize the access:
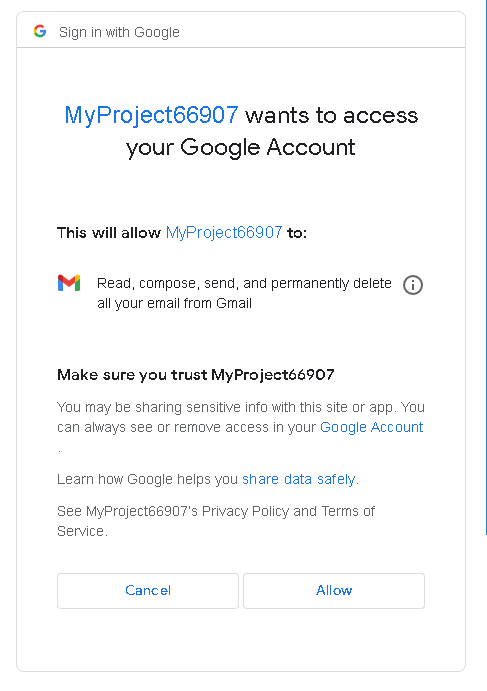
After this step user is redirected back to your website (http://www.yourdomain.com/oauth2callback). Following is this callback code. Its purpose is to get a refresh-token and an access-token:
WebServerClient consumer= new WebServerClient(server, clientID, clientSecret);
consumer.ClientCredentialApplicator =
ClientCredentialApplicator.PostParameter(clientSecret);
IAuthorizationState grantedAccess = consumer.ProcessUserAuthorization(null);
string accessToken = grantedAccess.AccessToken;
An access token is usually valid for a maximum of one hour, and allows you to access the user’s data. You also received a refresh token. A refresh token can be used to request a new access token once the previous expired.
Access IMAP/SMTP server
Finally we’ll ask Google for user’s email and use LoginOAUTH2 method to access Gmail’s IMAP server:
GoogleApi api = new GoogleApi(accessToken);
string user = api.GetEmail();
using (Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.LoginOAUTH2(user, accessToken);
imap.SelectInbox();
List<long> uids = imap.Search(Flag.Unseen);
foreach (long uid in uids)
{
var eml = imap.GetMessageByUID(uid);
IMail email = new MailBuilder().CreateFromEml(eml);
Console.WriteLine(email.Subject);
}
imap.Close();
}
Refreshing access token
An access token is usually short lived and valid for a maximum of one hour. The main reason behind this is security and prevention of replay attacks. This means that for long-lived applications you need to refresh the access token.
In most cases web applications don’t need to refresh access token (they request new one every time), thus when using WebServerClient refresh token is not sent. To force sending refresh token you need to set access_type
url parameter to offline
:
AuthorizationServerDescription authServer = new AuthorizationServerDescription
{
AuthorizationEndpoint =
new Uri("https://accounts.google.com/o/oauth2/auth?access_type=offline"),
...
};
Your refresh token will be sent only once – don’t loose it!
We recommend storing entire IAuthorizationState object received from WebServerClient.ProcessUserAuthorization method call. This object contains both: refresh token and access token, along with its expiration time.
The process of refreshing access token is simple:
IAuthorizationState grantedAccess = ...
consumer.RefreshAuthorization(grantedAccess, TimeSpan.FromMinutes(20));
In the example above the access token will not be refreshed if its remaining lifetime exceeds 20 minutes.
Retrieving lost refresh token
When your application receives a refresh token, it is important to store that refresh token for future use. If your application loses the refresh token, it will have to re-prompt the user for consent before obtaining another refresh token.
You’ll need to add approval_prompt=force
to your parameters:
AuthorizationServerDescription authServer = new AuthorizationServerDescription
{
AuthorizationEndpoint =
new Uri("https://accounts.google.com/o/oauth2/auth?access_type=offline&approval_prompt=force"),
...
};