Delete email permanently in Gmail
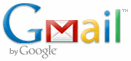
If you delete a message from your Inbox or one of your custom folders, it will still appear in [Gmail]/All Mail.
Here’s why: in most folders, deleting a message simply removes that folder’s label from the message, including the label identifying the message as being in your Inbox.
[Gmail]/All Mail shows all of your messages, whether or not they have labels attached to them.
If you want to permanently delete a message from all folders:
- Move it to the [Gmail]/Trash folder (or [Gmail]/Bin or national version).
- Delete it from the [Gmail]/Trash folder (or [Gmail]/Bin or national version).
All emails in [Gmail]/Spam and [Gmail]/Trash are deleted after 30 days.
If you delete a message from [Gmail]/Spam or [Gmail]/Trash, it will be deleted permanently.
Here’s how this looks like using Mail.dll .NET IMAP component:
Delete emails permanently (C# version)
// C# version:
using(Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("user@gmail.com", "password");
// Recognize Trash folder
List<FolderInfo> folders = imap.GetFolders();
CommonFolders common = new CommonFolders(folders);
FolderInfo trash = common.Trash;
// Find all emails we want to delete
imap.SelectInbox();
List<long> uids = imap.Search(
Expression.Subject("email to delete"));
// Move email to Trash
List<long> uidsInTrash = new List<long>();
foreach (long uid in uids)
{
long uidInTrash = (long)imap.MoveByUID(uid, trash);
uidsInTrash.Add(uidInTrash);
}
// Delete moved emails from Trash
imap.Select(trash);
foreach (long uid in uidsInTrash)
{
imap.DeleteMessageByUID(uid);
}
imap.Close();
}
Delete emails permanently (VB.NET version)
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("user@gmail.com", "password")
' Recognize Trash folder
Dim folders As List(Of FolderInfo) = imap.GetFolders()
Dim common As CommonFolders = new CommonFolders(folders)
Dim trash As FolderInfo = common.Trash
' Find all emails we want to delete
imap.SelectInbox()
Dim uids As List(Of Long) = imap.Search( _
Expression.Subject("email to delete"))
' Move email to Trash
Dim uidsInTrash As New List(Of Long)
For Each uid As Long In uids
Dim uidInTrash As Long = imap.MoveByUID(uid,trash)
uidsInTrash.Add(uidInTrash)
Next
' Delete moved emails from Trash
imap.[Select](trash)
For Each uid As Long In uidsInTrash
imap.DeleteMessageByUID(uid)
Next
imap.Close()
End Using
You can also use bulk methods to handle large amount of emails faster:
Delete emails permanently in bulk (C# version)
using(Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("user@gmail.com", "password");
// Recognize Trash folder
List<FolderInfo> folders = imap.GetFolders();
CommonFolders common = new CommonFolders(folders);
FolderInfo trash = common.Trash;
// Find all emails we want to delete
imap.SelectInbox();
List<long> uids = imap.Search(
Expression.Subject("email to delete"));
// Move emails to Trash
List<long> uidsInTrash = imap.MoveByUID(uids, trash);
// Delete moved emails from Trash
imap.Select(trash);
imap.DeleteMessageByUID(uidsInTrash);
imap.Close();
}
Delete emails permanently in bulk (VB.NET version)
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("user@gmail.com", "password")
' Recognize Trash folder
Dim folders As List(Of FolderInfo) = imap.GetFolders()
Dim common As CommonFolders = new CommonFolders(folders)
Dim trash As FolderInfo = common.Trash
' Find all emails we want to delete
imap.SelectInbox()
Dim uids As List(Of Long) = imap.Search( _
Expression.Subject("email to delete"))
' Move email to Trash
Dim uidsInTrash As List(Of Long) = imap.MoveByUID(uids, trash)
' Delete moved emails from Trash
imap.[Select](trash)
imap.DeleteMessageByUID(uidsInTrash)
imap.Close()
End Using
Please also note that there are 2 additional sections in the “Gmail settings”/”Forwarding and POP/IMAP” tab:
“When I mark a message in IMAP as deleted:”
- Auto-Expunge on – Immediately update the server. (default)
- Expunge off – Wait for the client to update the server.
“When a message is marked as deleted and expunged from the last visible IMAP folder:”
- Archive the message (default)
- Move the message to the Trash
- Immediately delete the message forever
Those settings change the default behavior of the account and modify DeleteMessage* methods behavior.
April 16th, 2014 at 12:51
Topic: deleting email permanently
long uidInTrash = (long)imap.MoveByUID(uid, “[Gmail]/Trash”);
from this line of code get the gollwing error:
Error 1 Cannot convert type ‘void’ to ‘long’
April 16th, 2014 at 14:22
@Pintu Make sure you have the latest version installed.
July 29th, 2014 at 10:41
How to do the same for POP3 accounts?
July 29th, 2014 at 11:39
@Farooq
POP3 protocol does not have a concept of seen/unseen emails nor folders.
If you need to delete an email just use Pop3.DeleteMessage or Pop3.DeleteMessageByUID method.
January 8th, 2015 at 17:57
Hi,
I like to delete my sent mails in gamil. how can i do this.
January 8th, 2015 at 18:31
@Vinay
Instead of
imap.SelectInbox()
use
imap.Select("[Gmail]/Sent Mail")
If you want to delete all emails in this folder replace the search code with:
List uids = imap.GetAll();
-or-
Dim uids As List(Of Long) = imap.GetAll()
January 9th, 2015 at 09:08
A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond 74.125.155.109:143
I am getting this error.Can you please tell me how to fix this.
January 9th, 2015 at 12:42
@Vinay
Most likely your server does not require SSL. Just use Imap.Connect method in that case. Please also read this post: Connection attempt failed.
Please contat us directly if you have other connection problems.
April 19th, 2015 at 10:49
hi,
i want to delete mails from outlook.
plz Ans,.
thank you.
April 20th, 2015 at 06:35
@hiremath
With Mail.dll you can delete and manage email on any IMAP or POP3 server (including Exchange).
Outlook is a client application. If you want to delete email from a local pst store, Mail.dll is not going to help you, but if you want to delete emails from Exchange read this post: Delete email messages using IMAP. You may need to establish SSL connection but it’s quite easy just use ConnectSSL method.
July 11th, 2015 at 09:34
hey, I was wondering, whether similar code can be used to immediately delete mails from ONE particular contact?
July 12th, 2015 at 11:50
@fireandhemlock
You just need to change the search code.
The following code searches for all email sent from alice:
Read more about searching emails on IMAP server.
If you are using Gmail you also may also use Gmail’s specific search syntax (same as on Gmail web site).
April 15th, 2016 at 05:22
How can I erase an email from my company’s server when we use gmail but we have our own @xxxxx.org? This email was intended to be sent from my personal email. I commited the mistake using my droid gmail application. I need to erase this email permanently and right away from my company’s server. I ignore if they have flags or anything. I know the Techn. guy is really good at monitoring us. That kind of company unfortunately. THANKS!
April 15th, 2016 at 09:50
If the message was already sent there is nothing you can do to ‘revoke’ it. It has been relied to other SMTP servers and/or other users accounts on POP3/IMAP servers.
You can delete it from your ‘sent mail’ folder, but it will not delete it from recipients’ inboxes.
September 23rd, 2017 at 13:59
hi sir
for gmail is is [Gmail] (as in imap.[Select](“[Gmail]/Trash”)) what is mail box name for outlook.
September 23rd, 2017 at 17:36
@Santhosh,
For Outlook.com trash folder is called “Deleted”. You can use CommonFolders class to get known folders:
You can find more details under common IMAP folders.
January 25th, 2018 at 06:47
when i want to delete bulk mail list and i move that id’s to trash it gives empty list
List uidlistInTrash =new List();
uidlistInTrash =p_imapClient.MoveByUID(p_uidlist, “[Gmail]/Trash”);
here p_uidlist contains 15 items still it gives uidlistInTrash 0
February 2nd, 2018 at 15:45
@John,
Were those emails actually moved?
Could you please send us the logs (http://www.limilabs.com/blog/logging-in-mail-dll)
I’ve double checked and I’m absolutely sure that MoveByUID returns non-empty list in such case.