Download emails from Gmail
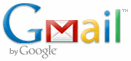
The task is quite easy with Mail.dll IMAP client. IMAP protocol unlike POP3 stores the unseen information on the server. So all we need to do is connect via SSL/TLS, search for unseen emails, and download them. Mail.dll will perform all the hard work.
First thing to remember is to enable IMAP in Gmail (your authentication options are app-passwords and OAuth 2.0) , second is to use ConnectSSL
method, as Gmail allows secure SSL/TLS connections only.
We’ll use Imap.Search(Flag.Unseen)
method to download unique ids of all unseen email messages in our inbox. Finally the sample shows how to download such emails and parse them using MailBuilder
class. After this it is easy to access any email data like: Subject
, Date
, From
and To
collections, plain-text and HTML versions of the email. Also all attachments are downloaded and stored in IMail.Attachments
collection.
// C# code:
using(Imap imap = new Imap())
{
imap.ConnectSSL("imap.gmail.com");
imap.UseBestLogin("pat@gmail.com", "app-password");
imap.SelectInbox();
Lis<long> uids = imap.Search(Flag.Unseen);
foreach (long uid in uids)
{
var eml = imap.GetMessageByUID(uid);
IMail mail = new MailBuilder().CreateFromEml(eml);
Console.WriteLine(mail.Subject);
Console.WriteLine(mail.Text);
}
imap.Close();
}
' VB.NET code:
Using imap As New Imap()
imap.ConnectSSL("imap.gmail.com")
imap.UseBestLogin("pat@gmail.com", "password")
imap.SelectInbox()
Dim uids As List(Of Long) = imap.Search(Flag.Unseen)
For Each uid As Long In uids
Dim eml = imap.GetMessageByUID(uid)
Dim mail As IMail = New MailBuilder().CreateFromEml(eml)
Console.WriteLine(mail.Subject)
Console.WriteLine(mail.Text)
Next
imap.Close()
End Using
If you like the idea of such simple Gmail access, just give it a try and download Mail.dll .NET IMAP component.
The next step is to learn how to process email attachments.
December 1st, 2013 at 15:54
[…] Mail.dll has of course IMAP support […]
November 15th, 2014 at 15:14
[…] articles can help you with that: How to enable POP3 for Gmail How to enable IMAP for Gmail How to enable IMAP for […]
October 21st, 2015 at 17:55
[…] Mail.dll contains ready to use IMAP client. Take a look at the IMAP sample. […]
August 4th, 2016 at 14:15
Id like to know how to keep messages in my GMAIL INBOX marked as UNREAD, using this procedure.
August 6th, 2016 at 06:41
@Matias,
You can use PeekMessageByUID method if you don’t want the message to be marked as seen after download.
August 27th, 2016 at 19:11
You may know the old read mail in GMAIL? I have to compare all the emails?
or I can run a query?
August 28th, 2016 at 09:23
@David
You can search for certain flags (seen, unseen),
you may also search using Gmail’s search syntax.
January 28th, 2017 at 11:23
How can I check my Gmail inbox?
January 29th, 2017 at 07:06
@Peace
What is your problem exactly?
April 9th, 2017 at 16:16
Does it is possible to mark the unseen mail as seen in gmail. That is once the unseen mail will be read then and then it will mark in gmail server. So that from next time always the unseen mails will be received.
April 9th, 2017 at 17:09
@Kaushik
You can either peek email on the server, by using one of the PeekXXX methods (e.g. PeekMessageByUID), as they don’t change the state of the message on the server
-or-
you can mark message seen or unseen explicitly.
June 20th, 2017 at 08:03
it doesn’t work for me
No connection could be made because the target machine actively refused it
June 20th, 2017 at 12:35
@Sravan
Sometimes firewall or antivirus software may block your connection attempt.
However most likely you provided incorrect server address and/or SSL usage. Gmail requires implicit SSL:
Remember to enable IMAP protocol access for your Gmail account.
If you are using different provider you might want to read this article:
http://www.limilabs.com/blog/connection-attempt-failed
September 23rd, 2017 at 07:24
hi sir
thank you
it works for me as i needed, but my other requirement is to move the read mails to a specified folder.
could you please help me here
Thanks in advance.
September 23rd, 2017 at 17:39
@Santhosh,
Simply use MoveByUID method or you can use GmailLabelMessageByUID (and GmailUnlabelMessageByUID) to label a message.
March 5th, 2018 at 00:43
Can i search only for all reply messages?
Meaning download only email that reply to my message.
March 5th, 2018 at 09:22
@Eve,
You can search IMAP using Expression.Header(“In-Reply-To”, “original message id”). If you are using Gmail you can get entire thread using Gmail thread id